React Fiber: Analysis, Operation, and Effects
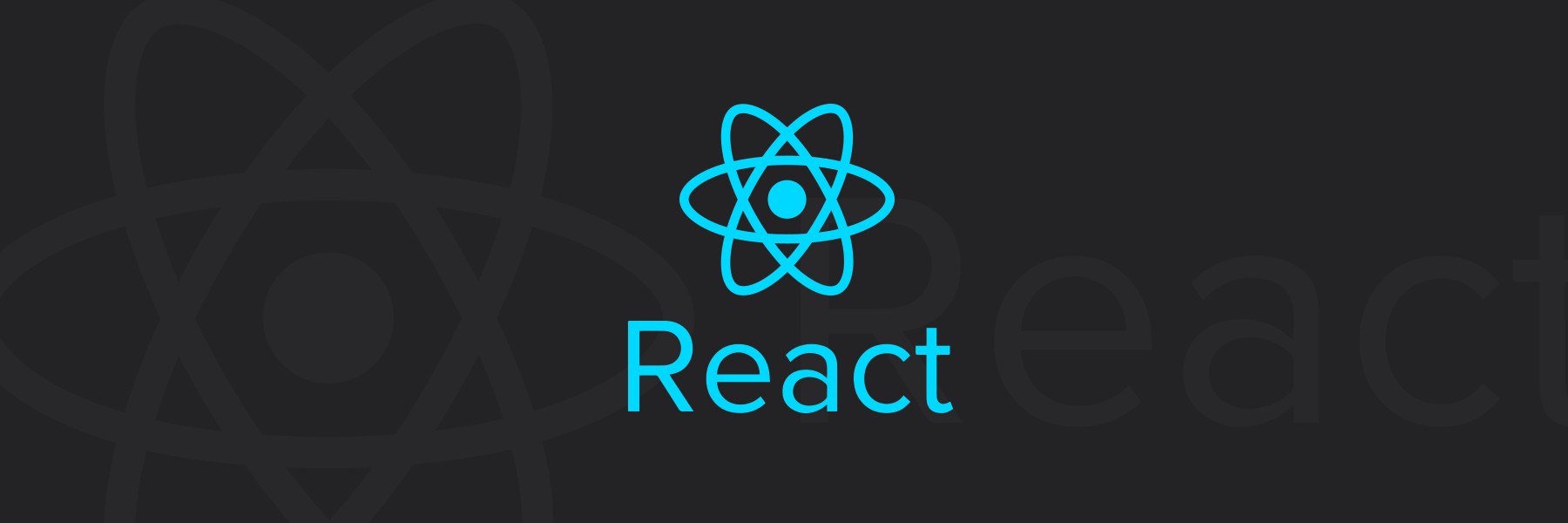
- Published on
- /2 mins read
How Fiber Works
Fiber optimizes the UI update process through three main phases:
Render Phase (Can be interrupted)
- Determines what changes need to be made.
- Traverses the Fiber tree using the Reconciliation algorithm.
- Compares the old and new Virtual DOM (diffing).
- Creates a list of changes (work-in-progress tree).
🛑 This phase can be interrupted if the browser needs to handle critical tasks like scrolling or animations.
Commit Phase (Cannot be interrupted)
- Applies changes from the Fiber tree to the actual DOM.
- Runs componentDidMount, componentDidUpdate, useEffect.
✅ Since React pre-calculates all changes in the Render Phase, the Commit Phase is very fast.
Work Loop & Scheduler
- React uses a scheduler to control execution time.
- The scheduler assigns priority levels to tasks.
- If there is a high-priority task (e.g., user interaction), React pauses the Render Phase and handles that task first.
- Uses mechanisms like requestIdleCallback or MessageChannel to optimize scheduling.
Effects and Benefits of Fiber
Feature | React Stack (Old) | React Fiber (New) |
---|---|---|
Performance | Blocking (synchronous) | Non-blocking (can pause) |
UI Updates | Entire component tree | Partial updates |
Animation & Gesture | Laggy when loading | Smoother, UI prioritized |
Concurrent Mode | Not supported | Supported (future) |
Error Handling | No Error Boundary | Supports Error Boundary |
Memory Usage | Higher | More optimized |
Real-world Applications of Fiber
- Suspense & Lazy Loading: Load components smoothly.
- Concurrent Mode: Enhances UI experience by prioritizing critical tasks.
- Better Animation: React can pause rendering to prioritize animations.
Conclusion
React Fiber makes React more powerful by optimizing UI updates, improving performance, and reducing lag in complex interfaces. This is a major advancement that helps React compete with other frameworks like Vue and Svelte. 🚀
← Previous postReact as Snapshot
Next post →Lifecycle of Reactive Effects